JavaScriptでUUID風のランダムな文字列とパスワード生成器を作る方法
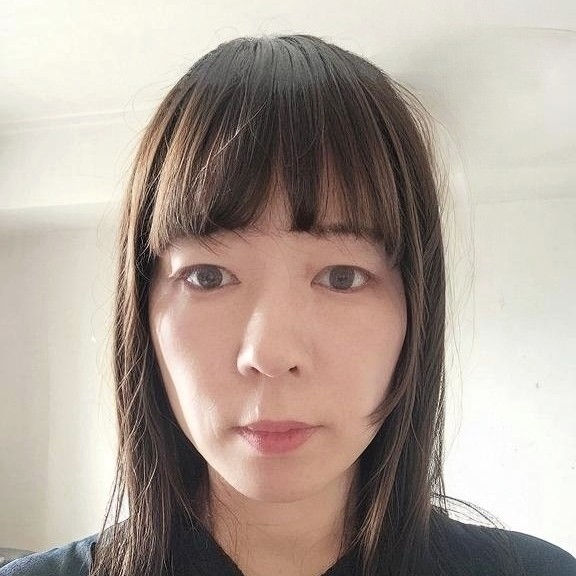
仲川 舞
ランダムな文字列は、アプリケーションの識別子や一意のトークン、セキュアなパスワードを生成する際に役立ちます。この記事では、JavaScriptでUUID風の文字列やランダムなパスワードを生成する方法について解説します。
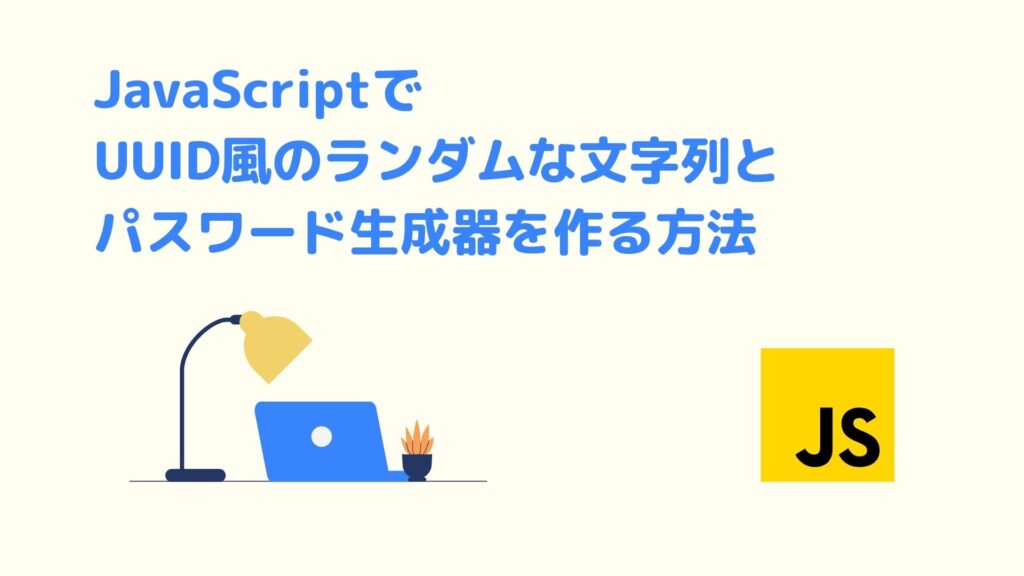
UUID風のランダム文字列を生成する
UUID(Universally Unique Identifier)は、一意性を持つ文字列を生成するための標準的な方法です。以下は、JavaScriptを使ったUUID風の文字列生成の例です。
ここにUUIDが表示されます
function generateUUID() {
return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(character) {
// 0〜15のランダムな整数値を生成
const randomValue = Math.random() * 16 | 0;
let generatedCharacter;
if (character === 'x') {
// 'x'にはランダムな値をそのまま使用
generatedCharacter = randomValue;
} else {
// 'y'は、UUIDの仕様に準拠した形に調整
generatedCharacter = (randomValue & 0x3) | 0x8;
}
return generatedCharacter.toString(16); // 16進数に変換して返す
});
}
console.log(generateUUID());
// 例: e3b0c442-98fc-1c14-9af9-8f12d9c6eafa
解説
'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'
文字列のテンプレートとしてUUIDの形式を指定します。/[xy]/g
文字列の中で、x
とy
の部分をランダムな値に置き換えます。Math.random()
ランダムな値を生成するために使用します。randomValue & 0x3 | 0x8
UUIDの仕様に準拠するためにy
部分のビットを調整しています。
応用: パスワード生成器を作成する
次に、特殊文字を含むランダムなパスワード生成器を作成してみます。
ここにパスワードが表示されます
<div class="password" id="randomPasswordOutput">ここにパスワードが表示されます</div>
<button onclick="generateAndDisplayPassword()">パスワード生成</button>
function generatePassword(length) {
const characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789!@#$%^&*()_+';
let password = '';
for (let i = 0; i < length; i++) {
const randomIndex = Math.floor(Math.random() * characters.length);
password += characters[randomIndex];
}
return password;
}
function generateAndDisplayPassword() {
const length = 12; // パスワードの文字数
const password = generatePassword(length);
document.getElementById('randomPasswordOutput').textContent = password;
}
解説
characters
パスワードに使用する文字のセット。大文字、小文字、数字、記号を含めています。Math.random()
配列からランダムなインデックスを取得するために使用します。password += characters[randomIndex]
文字列を1文字ずつランダムに追加していきます。
応用例: 特定の要件に対応するパスワード
例えば、必ず大文字、小文字、数字、特殊文字を含むパスワードを生成したい場合は、以下のように実装します。
ここにパスワードが表示されます
<div class="password" id="securePasswordOutput">ここにパスワードが表示されます</div>
<button onclick="generateAndDisplaySecurePassword()">パスワード生成</button>
function generateSecurePassword(length) {
const lower = 'abcdefghijklmnopqrstuvwxyz';
const upper = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ';
const numbers = '0123456789';
const symbols = '!@#$%^&*()_+';
const all = lower + upper + numbers + symbols;
let password = '';
// 各カテゴリから最低1文字を追加
password += lower[Math.floor(Math.random() * lower.length)];
password += upper[Math.floor(Math.random() * upper.length)];
password += numbers[Math.floor(Math.random() * numbers.length)];
password += symbols[Math.floor(Math.random() * symbols.length)];
// 残りの文字をランダムに追加
for (let i = 4; i < length; i++) {
password += all[Math.floor(Math.random() * all.length)];
}
// シャッフルしてランダム性を強化
return password.split('').sort(() => Math.random() - 0.5).join('');
}
function generateAndDisplaySecurePassword() {
const length = 12; // パスワードの文字数
const password = generateSecurePassword(length);
document.getElementById('securePasswordOutput').textContent = password;
}
まとめ
- UUID風の文字列生成は、テンプレートとランダム性を組み合わせて簡単に作成できます。
- ランダムパスワード生成器では、要件に応じて文字セットや生成ロジックをカスタマイズできます。